Create a smooth scroll to link in Webflow
Creating a smooth scroll animation when clicking on anchor links is pretty easy in Webflow with a little help of JavaScript code and custom webflow attributes.
The code that we’re going to write will use attributes that are set inside Webflow on multiple links. First we add a custom attribute to the anchor link with the Name data-scrollto
and a value of true
.
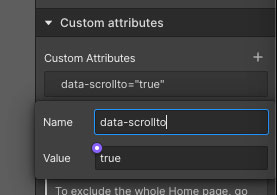
Make sure the link element also has a hash link with the ID set to the ID os the section we want to smoothly scroll to. In our case the section ID is #section-1
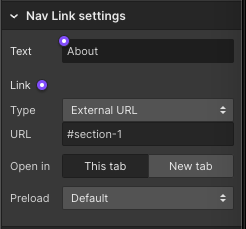
After that we can copy the JS code either to the page custom code or even better the site wide custom code, so it’s available on the whole site on every page.
document.addEventListener("DOMContentLoaded", function () {
// Select all the links that have the custom attribute
const scrollLinks = document.querySelectorAll("[data-scrollto='true']");
function handleScrollToClick(event) {
event.preventDefault();
// select the value from the href attribute
let targetId = this.getAttribute("href");
// Check if it starts with "#" and remove it
if (targetId && targetId.startsWith("#")) {
targetId = targetId.slice(1); // Remove the "#" character
}
// Select the target element with the ID from href attribute
const targetElement = document.getElementById(targetId);
const duration = 500; // Adjust the duration as needed
if (targetElement) {
// Scroll to the target section smoothly
const targetOffset =
targetElement.getBoundingClientRect().top + window.scrollY;
const startingY = window.scrollY;
const startTime = performance.now();
function scrollStep(timestamp) {
const progress = (timestamp - startTime) / duration;
if (progress < 1) {
window.scrollTo(0, startingY + (targetOffset - startingY) * progress);
requestAnimationFrame(scrollStep);
} else {
window.scrollTo(0, targetOffset);
}
}
requestAnimationFrame(scrollStep);
}
}
if (scrollLinks.length) {
// Apply event listeners to all the links with the custom attribute
scrollLinks.forEach(function (scrollLink) {
scrollLink.addEventListener("click", handleScrollToClick);
});
}
});
Now when we publish the site, clicking on the link should smoothly take us to the section with the specified ID. The script above takes the href value from the anchor link and uses it to find the section with the ID on the page.